High speed hypermedia-driven REST API development with Spring Data REST
This post briefly documents the process of rapidly developing a hypermedia-driven REST web service.
Introduction
Spring Data REST is part of the umbrella Spring Data project. It builds on top of Spring Data repositories, analyzes your application’s domain model and exposes hypermedia-driven HTTP resources for aggregates contained in the model.
HAL Explorer lets you easily explore HAL and HAL-FORMS based HTTP responses. It also supports Spring profiles generated by Spring Data REST. You can point it at any Spring Data REST API and use it to navigate the app and create new resources.
Features
The following features are supported out of the box and no additional coding is necessary.
- Pagination & Sorting
- Exposes a discoverable REST API for your domain model using HAL as media type
Minimum Software Requirements
- Java
Sample Project
Spring Data Rest is the sample Spring Boot application i've used to illustrate the usage of the aforementioned tool.
Navigate to http://localhost:8080/api/v1 to discover the application URLs via HAL Explorer.
Noticed an issue with this Sample Project? Open an issue or a PR on GitHub!
Dependencies
This implementation has one main dependency, Rest Repositories. Optionally, you can add Rest Repositories HAL explorer to serve as an interactive documentation provider. The maven/gradle dependencies of the same are mentioned below.
Rest Repositories
<!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-data-rest -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-rest</artifactId>
<version>2.6.6</version>
</dependency>
or
// https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-data-rest
implementation group: 'org.springframework.boot', name: 'spring-boot-starter-data-rest', version: '2.6.6'
Rest Repositories HAL explorer
<!-- https://mvnrepository.com/artifact/org.springframework.data/spring-data-rest-hal-explorer -->
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-rest-hal-explorer</artifactId>
<version>3.6.3</version>
</dependency>
or
// https://mvnrepository.com/artifact/org.springframework.data/spring-data-rest-hal-explorer
implementation group: 'org.springframework.data', name: 'spring-data-rest-hal-explorer', version: '3.6.3'
API Base Path & Page Size
In the application-h2db.properties file configure the following key-values.
# Base path to be used by Spring Data REST to expose repository resources.
spring.data.rest.base-path=/api/v1
# Default size of pages.
spring.data.rest.default-page-size=25
Basic Usage
- Define entities as you would in any Java/Spring-Boot project.
- Create a repository interface for the defined entities except this time around, instead of anotating the interface with @Repository anotation, use @RepositoryRestResource() anotation.
That's it, the application now exposes discoverable REST API with out of the box support for pagination and sorting for your domain models.
HAL Explorer
- Visual Documentation via HAL Explorer : http://localhost:8080/api/v1/explorer
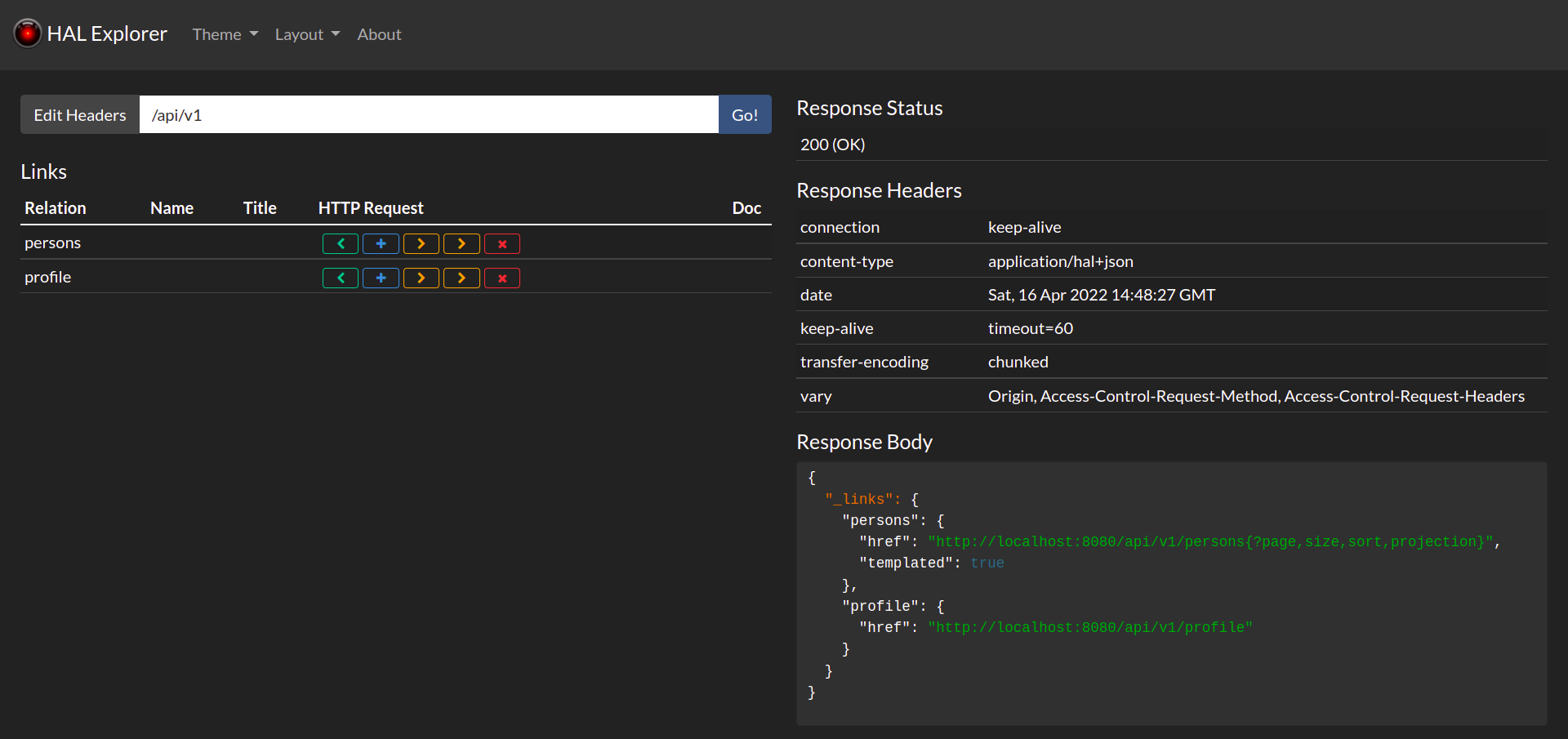
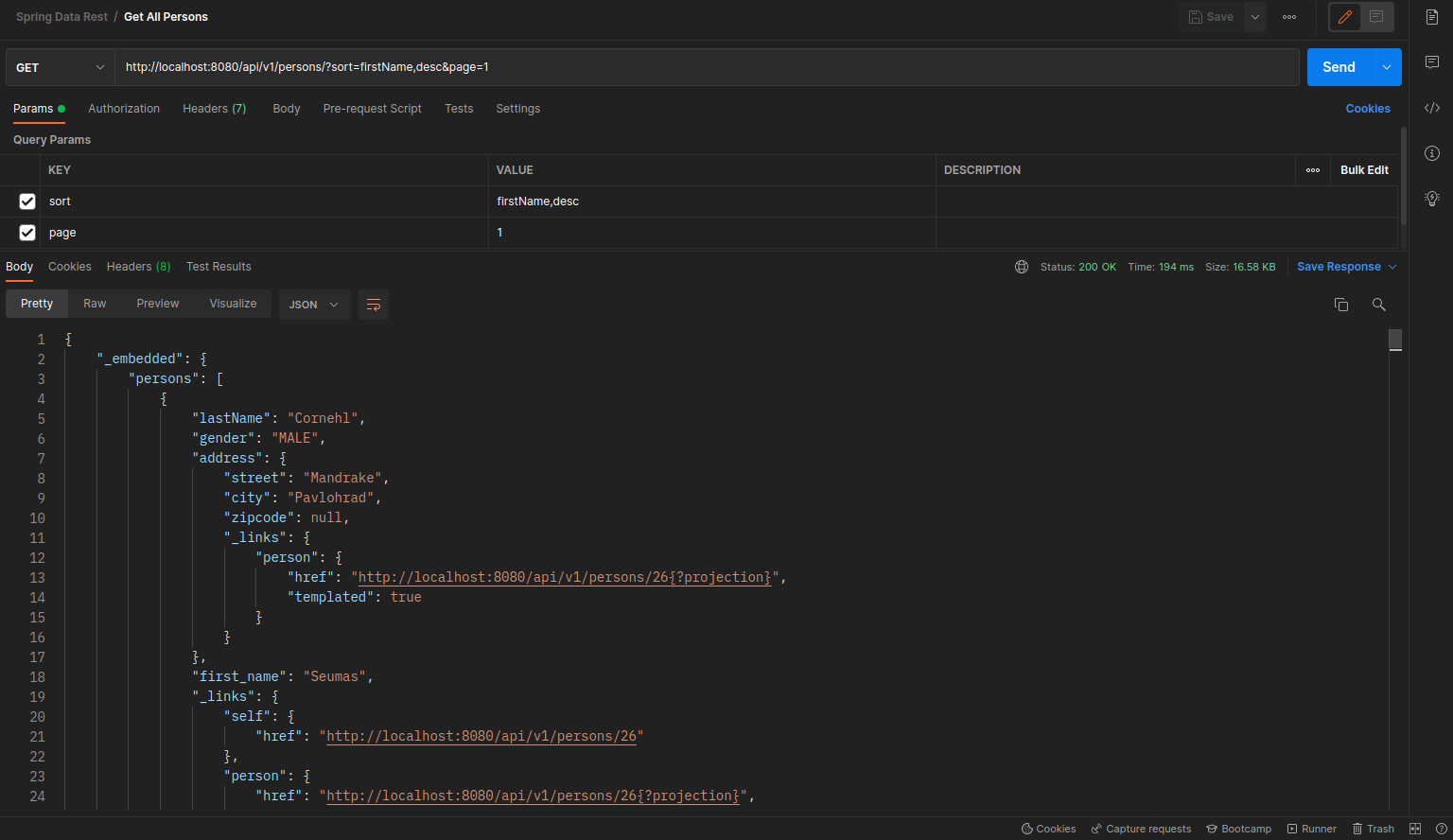
In order to expose the id of the resource, create a configuration class that implements RepositoryRestConfigurer.
Override the configureRepositoryRestConfiguration(RepositoryRestConfiguration config, CorsRegistry cors) method and pass the domain classes for which you want to expose the id's.
Projections
Spring Data REST presents a default view of the domain model you export. However, sometimes, you may need to alter the view of that model for various reasons. This section covers how to define projections to serve up simplified and reduced views of resources.
Create an interface and add only the variables that you'd like to serve. Anotate this interface with @Projection. Name it and add the type whose variables are being served.
Next, when requesting for the resources, pass the name of the projection as the Query Parameter
example: http://localhost:8080/api/v1/persons/1?projection=idAndFullName
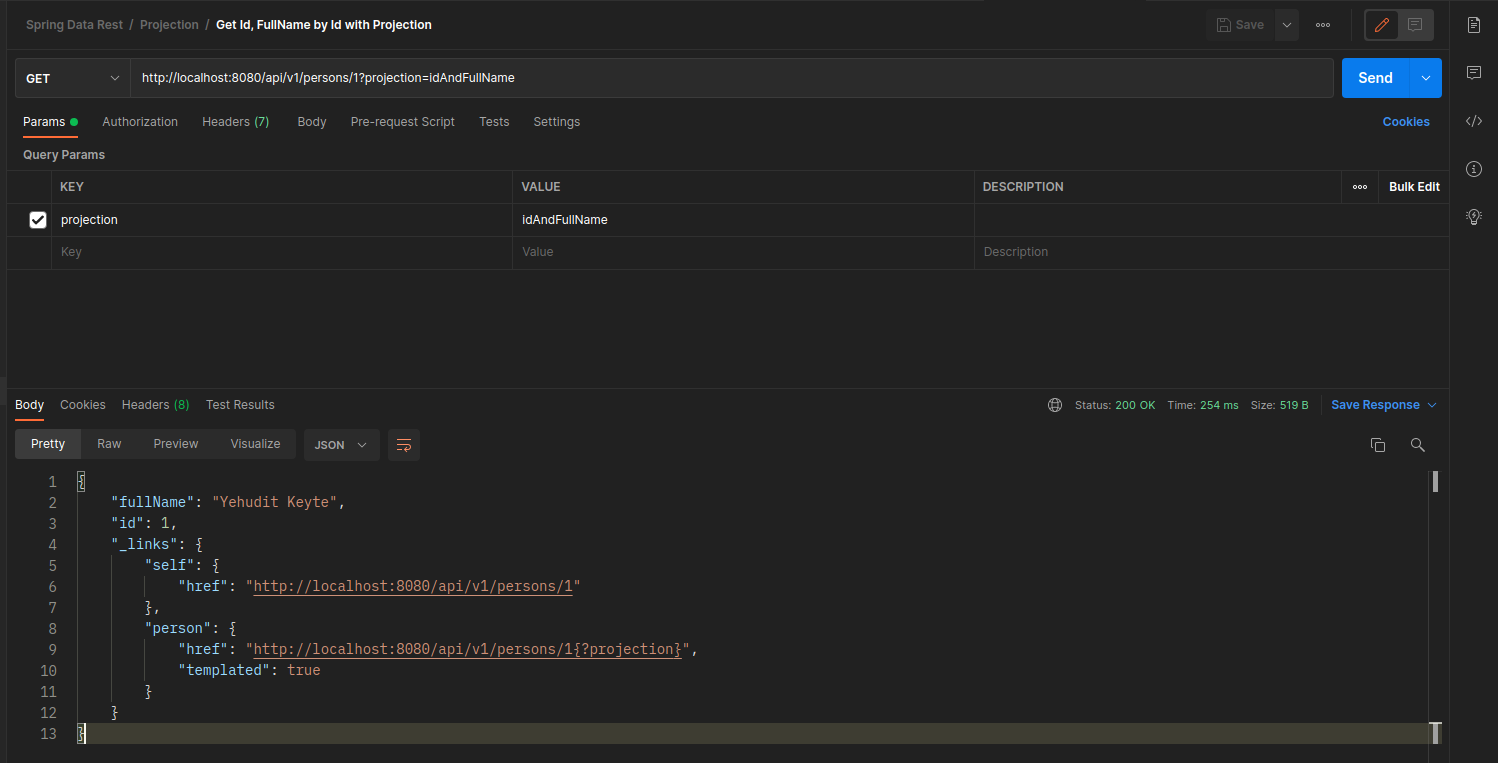
References
HAL Explorer Source Code
HAL Explorer Documentation
Spring Data REST
Spring Data REST Reference Guide